在开发过程中,为了方便我们可能会将所有的代码都放在一个项目中,所有人的工作内容都集中在一起。随着业务的快速发展,会导致整个项目越来越复杂和后期维护难等问题。而项目模块化可以更好的实现代码复用,简化项目结构,使逻辑更加清晰,也方便团队分工合作,后期扩展和维护。
本文将以一个个人博客项目为例,简单讲解如何搭建一个基于Spring Boot
的Maven
多模块项目。
环境:
- JDK
- Maven(3.3.9)
- Intellij IDEA
1. 首先创建一个Spring Boot
父项目
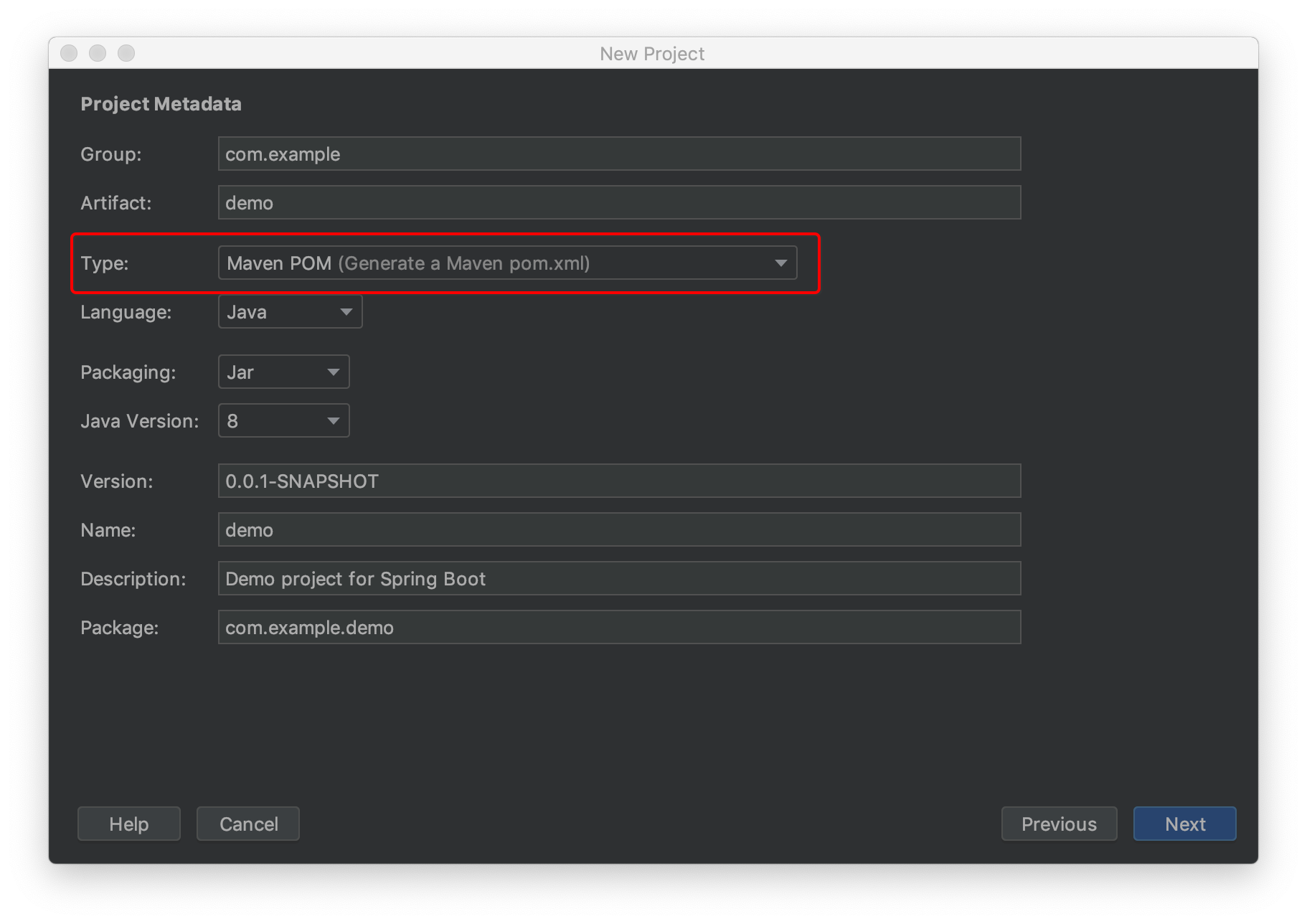
注意Type
类型选择pom
项目结构如下图所示
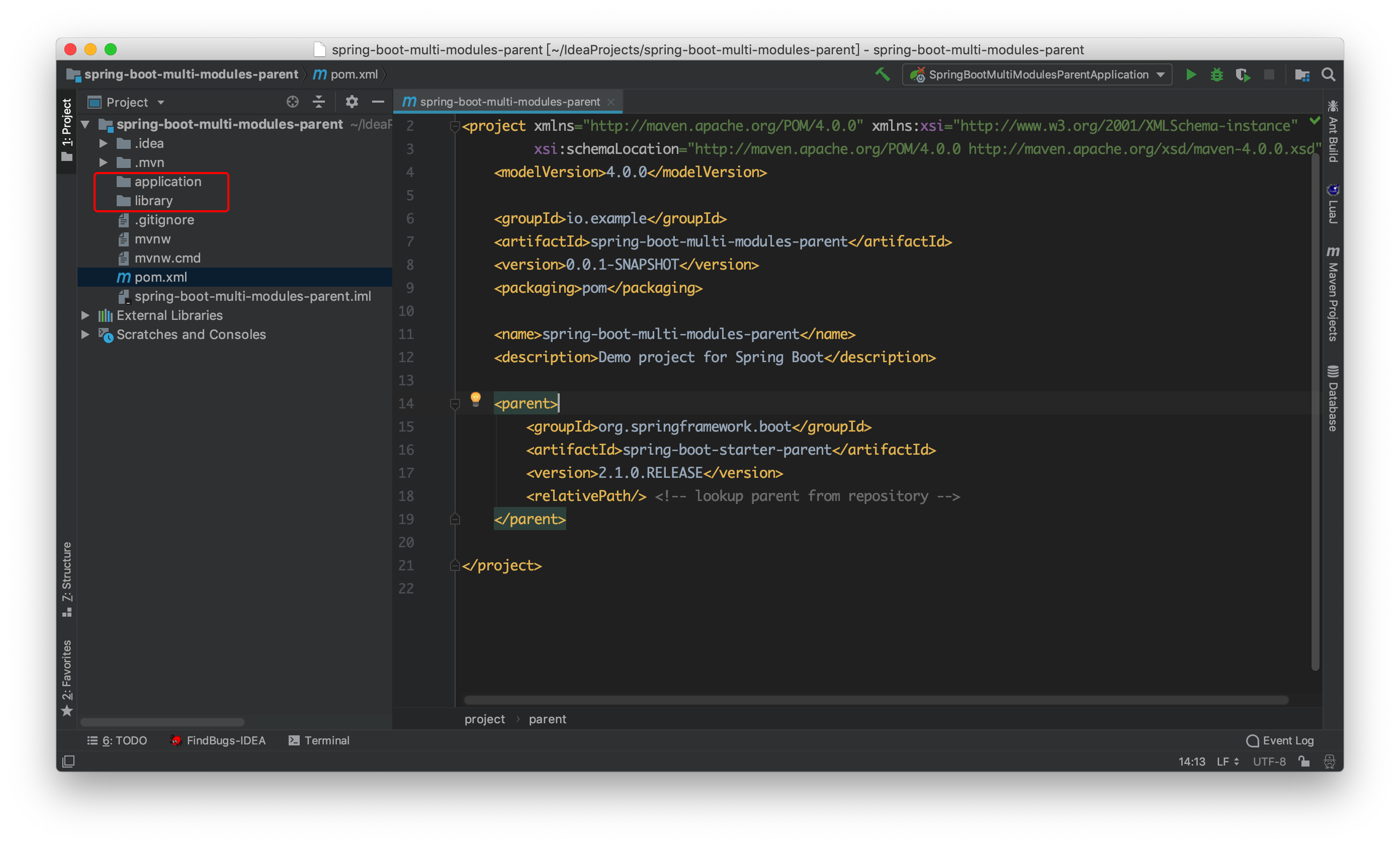
pom
配置文件修改成如图所示。图中标出的两个文件夹是用来存放子项目,library
用来存放依赖的项目,application
用来存放应用,当然也可以选择直接放在根目录。
2. 创建子项目
右键父项目,选择New
,然后选择Module
,注意选择Maven
类型项目
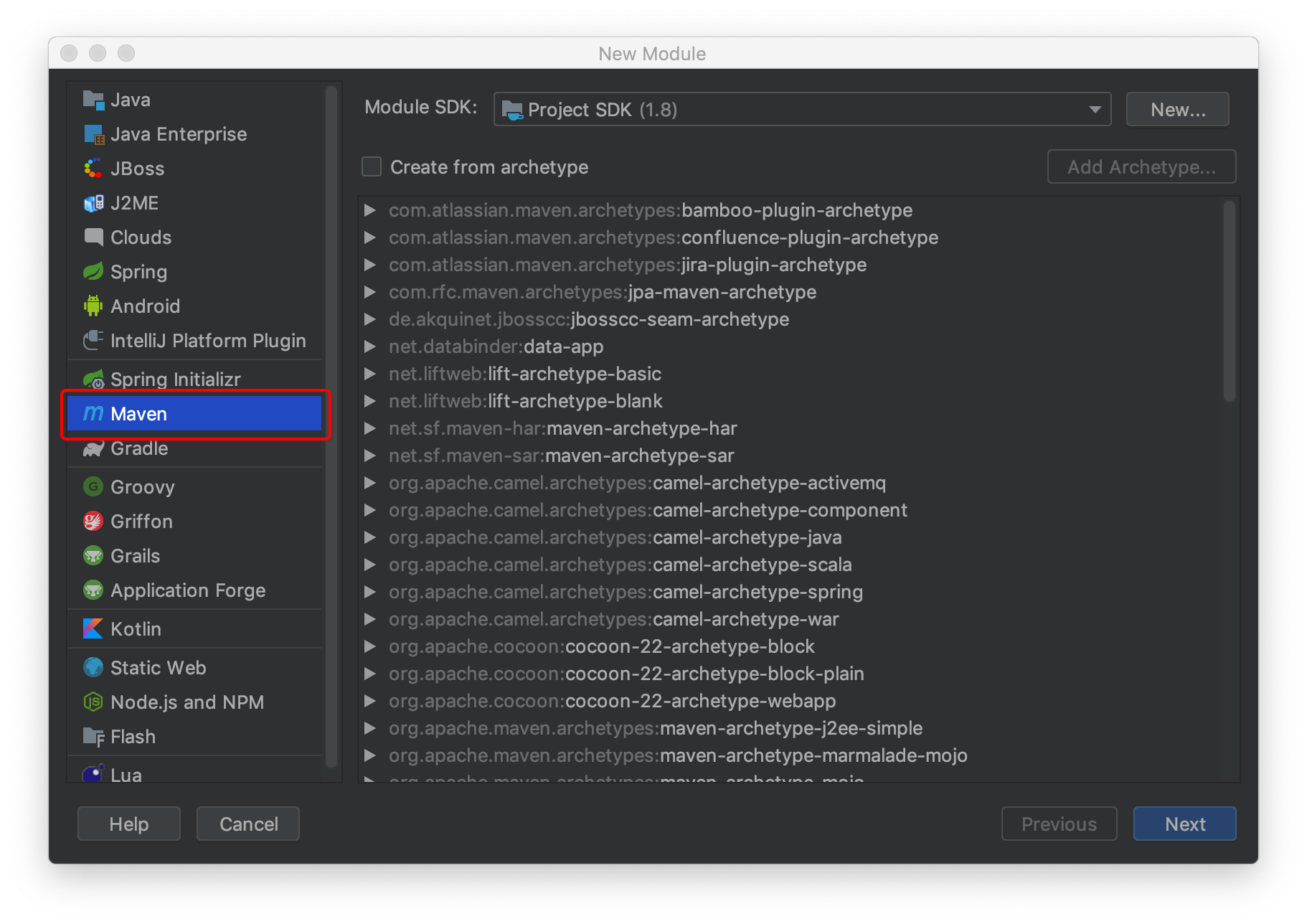
继续下一步
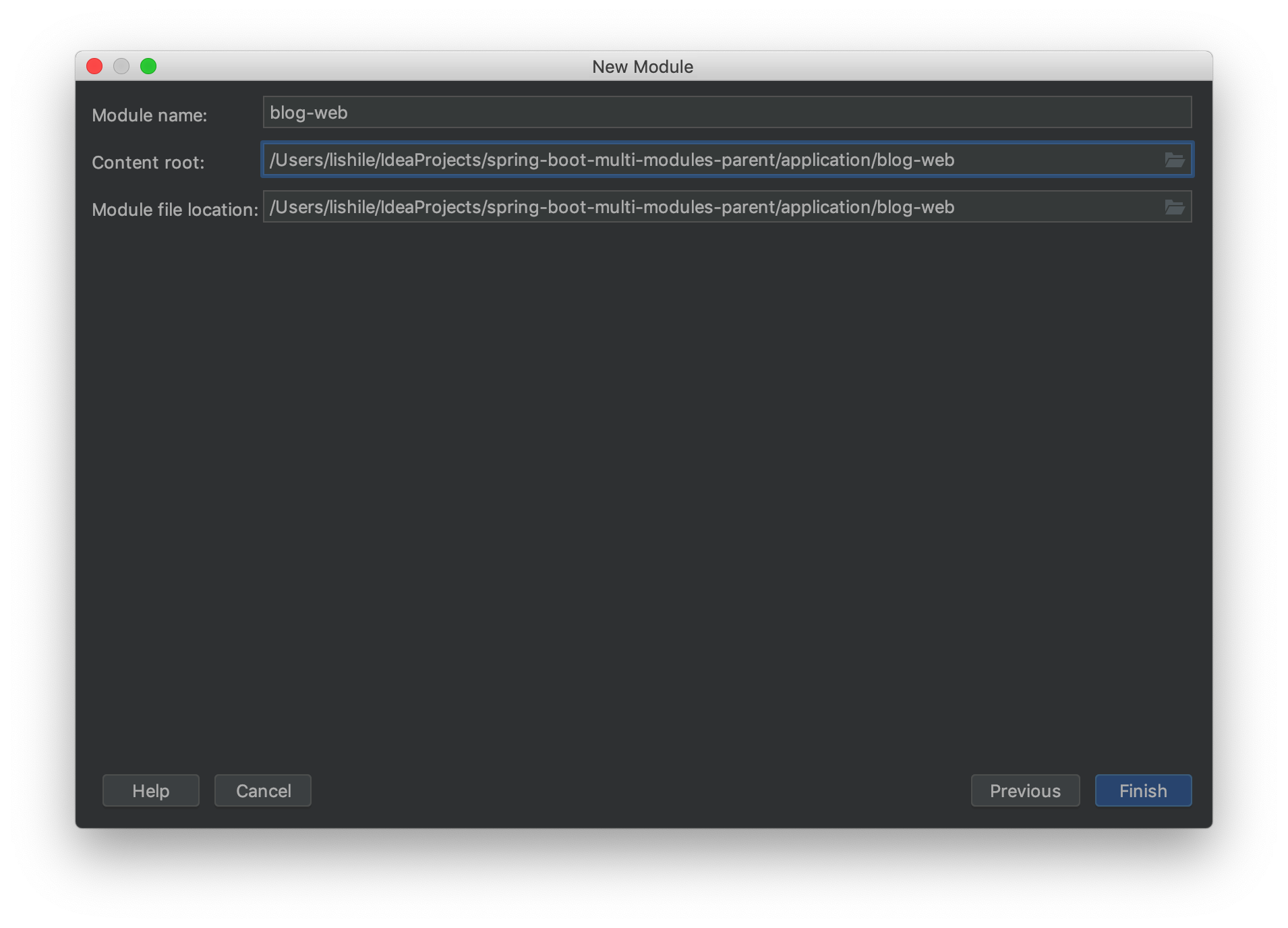
这里把blog-web
项目放在了application
文件夹,可以选择放在根目录,没有影响。
创建好直接项目结构如下
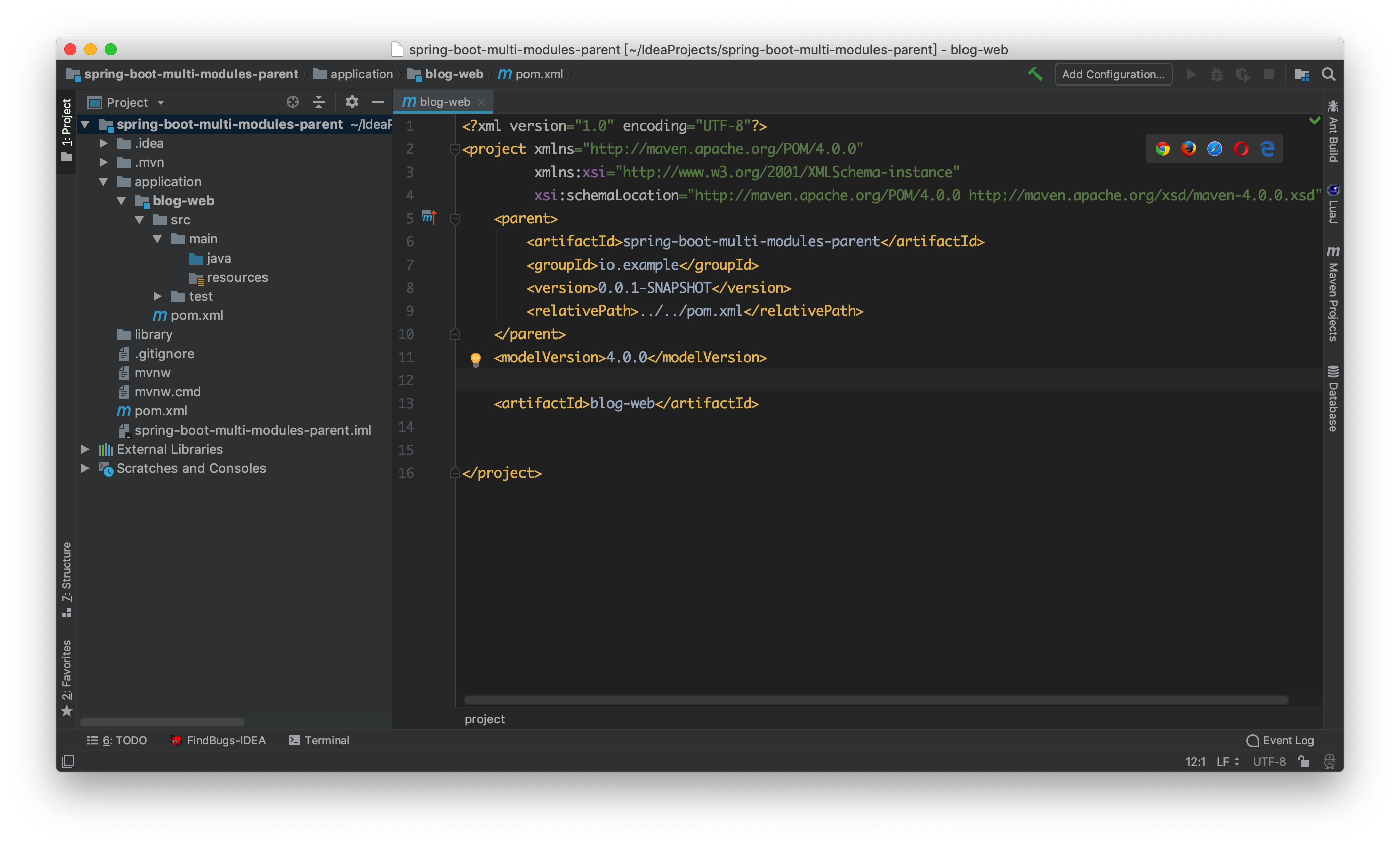
由于这里当前项目放在application
文件夹内,所以blog-web
中pom
文件中的relativePath
的配置如图所示。若子项目放在根目录,则配置略有不同,应该是../pom.xml
。
修改blog-web
的pom
文件
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45
| <?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <parent> <artifactId>spring-boot-multi-module</artifactId> <groupId>io.example</groupId> <version>0.0.1-SNAPSHOT</version> <relativePath>../../pom.xml</relativePath> </parent> <modelVersion>4.0.0</modelVersion>
<artifactId>blog-web</artifactId>
<dependencies> <dependency> <groupId>io.example</groupId> <artifactId>blog-service</artifactId> <version>0.0.1-SNAPSHOT</version> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency>
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> </dependency> </dependencies>
<build> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> </plugins> </build>
</project>
|
接下来依次创建service
和dao
模块,具体过程就不一一解释了,流程和上面叙述的相同,主要看下每个项目的pom
文件的配置。
blog-service
的pom
文件
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32
| <?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <parent> <artifactId>spring-boot-multi-module</artifactId> <groupId>io.example</groupId> <version>0.0.1-SNAPSHOT</version> <relativePath>../../pom.xml</relativePath> </parent> <modelVersion>4.0.0</modelVersion>
<artifactId>blog-service</artifactId>
<dependencies> <dependency> <groupId>io.example</groupId> <artifactId>blog-dao</artifactId> <version>0.0.1-SNAPSHOT</version> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter</artifactId> </dependency>
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> </dependency> </dependencies>
</project>
|
blog-dao
的pom
文件
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39
| <?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <parent> <artifactId>spring-boot-multi-module</artifactId> <groupId>io.example</groupId> <version>0.0.1-SNAPSHOT</version> <relativePath>../../pom.xml</relativePath> </parent> <modelVersion>4.0.0</modelVersion>
<artifactId>blog-dao</artifactId>
<dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-jpa</artifactId> </dependency> <dependency> <groupId>com.h2database</groupId> <artifactId>h2</artifactId> </dependency>
<dependency> <groupId>org.projectlombok</groupId> <artifactId>lombok</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> </dependency> </dependencies>
</project>
|
项目成功运行!!!
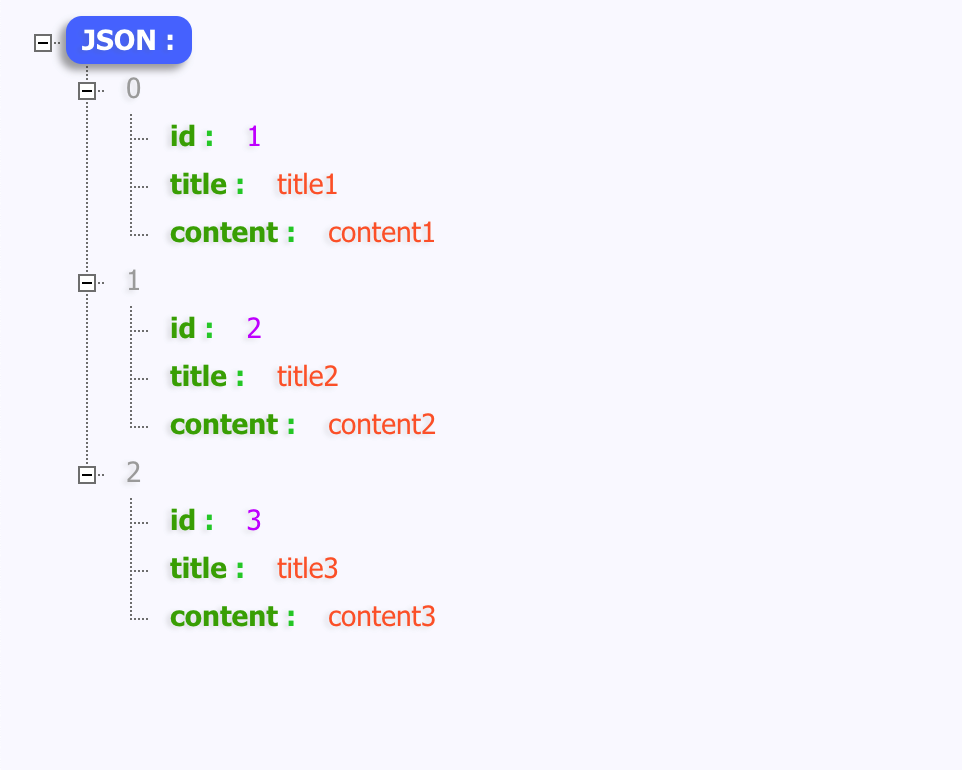
完整代码在GitHub上,有需要的同学可以自行下载。
注意:blog-dao
的application.yml
文件放在classpath:config
下面,是为了避免配置被覆盖,导致读取不到相关配置的问题。